Dialog styles
A detailed list of dialog styles can be found here. This page describes the behavior of ShowPlayerDialog and OnDialogResponse. For various limitations, visit the Limits page. The following debug output will be used in the examples listed on this page:
public OnDialogResponse( playerid, dialogid, response, listitem, inputtext[ ] ) { printf( "playerid = %d, dialogid = YOUR_DIALOGID, response = %d, listitem = %d, inputtext = '%s' (size: %d)", playerid, response, listitem, inputtext, strlen( inputtext ) ); return 1; }
- In OnDialogResponse, pressing button1 sets response to 1, while pressing button2 sets response to 0.
- Every dialog can have an optional button 2. To make it disappear leave it empty, like in the first example. Players won't be able to click it, but they will be able to press ESC and trigger OnDialogResponse with response = 0.
- ShowPlayerDialog: Color embedding can be used for every string: caption, info, button1 and button2.
Style 0: DIALOG_STYLE_MSGBOX
This style is pretty self-explanatory. It primarily displays a dialog with a message on it.
Screenshot
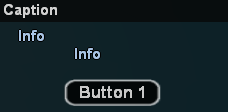
Creating the dialog
The following should be noted when using this dialog style:
- \t adds a TAB (more space).
- \n creates a new line.
- Color embedding won't reset after \n or \t.
ShowPlayerDialog(playerid, YOUR_DIALOGID, DIALOG_STYLE_MSGBOX, "Caption", "Info\n\tInfo", "Button 1", "");
Response output
- listitem is always -1.
- inputtext is always empty.
// pressed the button playerid = 0, dialogid = YOUR_DIALOGID, response = 1, listitem = -1, inputtext = '' (size: 0) // pressed ESC (as the second button isn't visible) playerid = 0, dialogid = YOUR_DIALOGID, response = 0, listitem = -1, inputtext = '' (size: 0)
Style 1: DIALOG_STYLE_INPUT
This dialog style can be used to display simple form fields and to get their input.
Screenshot

Creating the dialog
The following should be noted when using this dialog style:
- \t adds a TAB (more space).
- \n creates a new line.
- Color embedding won't reset after \n or \t.
ShowPlayerDialog(playerid, YOUR_DIALOGID, DIALOG_STYLE_INPUT, "Caption", "Enter information below:", "Button 1", "Button 2");
Response output
- listitem is always -1.
- inputtext is the text written by the user, including colors.
// wrote "input" and pressed the left button playerid = 0, dialogid = YOUR_DIALOGID, response = 1, listitem = -1, inputtext = 'input' (size: 5) // wrote "input" and pressed the right button playerid = 0, dialogid = YOUR_DIALOGID, response = 0, listitem = -1, inputtext = 'input' (size: 5)
Style 2: DIALOG_STYLE_LIST
This dialog style can be used for menus where there might be multiple options.
Screenshot

Creating the dialog
The following should be noted when using this dialog style:
- \t adds a TAB (more space).
- \n creates a new list item.
- Color embedding resets only after \n.
ShowPlayerDialog(playerid, YOUR_DIALOGID, DIALOG_STYLE_LIST, "Caption", "Item 0\n{FFFF00}Item 1\nItem 2", "Button 1", "Button 2");
Response output
- listitem is the number of the selected item, starting from 0.
- inputtext is the text contained by the selected listitem, without the colors.
// selected the first list item and pressed the left button playerid = 0, dialogid = YOUR_DIALOGID, response = 1, listitem = 0, inputtext = 'Item 0' (size: 6) // selected the second list item and pressed the right button playerid = 0, dialogid = YOUR_DIALOGID, response = 0, listitem = 1, inputtext = 'Item 1' (size: 6)
Style 3: DIALOG_STYLE_PASSWORD
This dialog style is very similar to DIALOG_STYLE_INPUT, but is instead designed for passwords.
Screenshot

Creating the dialog
The following should be noted when using this dialog style:
- \t adds a TAB (more space).
- \n creates a new line.
ShowPlayerDialog(playerid, YOUR_DIALOGID, DIALOG_STYLE_PASSWORD, "Caption", "Enter private information below:", "Button 1", "Button 2");
Response output
- listitem is always -1.
- inputtext is the text written by the user, not censored, including colors.
// wrote "input" and pressed the left button playerid = 0, dialogid = YOUR_DIALOGID, response = 1, listitem = -1, inputtext = 'input' (size: 5) // wrote "input" and pressed the right button playerid = 0, dialogid = YOUR_DIALOGID, response = 0, listitem = -1, inputtext = 'input' (size: 5)
Style 4: DIALOG_STYLE_TABLIST
This dialog style is very similar to DIALOG_STYLE_LIST, however this type can be used for tables.
Screenshot
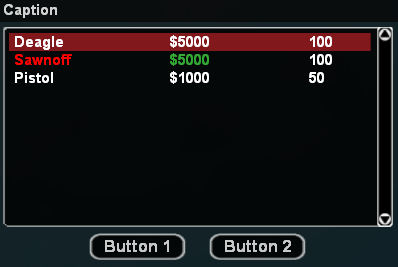
Creating the dialog
The following should be noted when using this dialog style:
- \t creates a new column.
- \n creates a new list item.
- Color embedding resets after \t and \n.
- The first info row contains the header.
ShowPlayerDialog(playerid, YOUR_DIALOGID, DIALOG_STYLE_TABLIST, "Caption", "Deagle\t$5000\t100\n\ {FF0000}Sawnoff\t{33AA33}$5000\t100\n\ Pistol\t$1000\t50", "Button 1", "Button 2");
Response output
- listitem is the number of the selected item, starting from 0.
- inputtext is the text contained by the first column of the selected listitem, without the colors.
// selected the first list item and pressed the left button playerid = 0, dialogid = YOUR_DIALOGID, response = 1, listitem = 0, inputtext = 'Deagle' (size: 6) // selected the second list item and pressed the right button playerid = 0, dialogid = YOUR_DIALOGID, response = 0, listitem = 1, inputtext = 'Sawnoff' (size: 7)
Style 5: DIALOG_STYLE_TABLIST_HEADERS
This dialog style is very similar to DIALOG_STYLE_LIST, however this type can be used for tables.
Screenshot

Creating the dialog
The following should be noted when using this dialog style:
- \t creates a new column.
- \n creates a new list item.
- Color embedding resets after \t and \n.
- The first info row contains the header.
ShowPlayerDialog(playerid, YOUR_DIALOGID, DIALOG_STYLE_TABLIST_HEADERS, "Caption", "Header 1\tHeader 2\tHeader 3\n\ Item 1 Column 1\tItem 1 Column 2\tItem 1 Column 3\n\ {FF0000}Item 2 Column 1\t{33AA33}Item 2 Column 2\tItem 2 Column 3", "Button 1", "Button 2");
Response output
- listitem is the number of the selected item, starting from 0.
- inputtext is the text contained by the first column of the selected listitem, without the colors.
// selected the first list item and pressed the left button playerid = 0, dialogid = YOUR_DIALOGID, response = 1, listitem = 0, inputtext = 'Item 1 Column 1' (size: 15) // selected the first list item and pressed the right button playerid = 0, dialogid = YOUR_DIALOGID, response = 0, listitem = 1, inputtext = 'Item 2 Column 1' (size: 15)