Colour list
Colours are used everywhere in UG-MP - from vehicles, player names and blips, textdraws, game text, and more. This page contains information on how to use them.
Table of contents
-
Chat text and player colour
- Alpha values (transparency)
- Doing math
- Convert string to value with pawn
-
Colour embedding
- Example
- Another example
- Using GetPlayerColor
- Colour pickers
- GameText
-
Vehicle & ped colours
-
Working with IDs
- Example
-
Working with RGB values
- Switching RGB colours on and off
- Changing the RGB colour on vehicles
-
Working with IDs
- Extra weathers
Chat text and player colour
Colours in SA-MP are generally represented in hexadecimal notation (though integers can be used also). A chattext or player color looks like this: 0xRRGGBBAA.
RR is the red part of the color, GG the green and BB the blue. AA is the alpha value. If FF is used there, the color will display without transparency and if 00 is used, it will be invisible.
Alpha values (transparency)
The following images display the effect of transparency values used with a white square under the player marker and left to the saving floppy icon. Increments of 0x11 (decimal 17) are used for demonstration, but of course you can use any value.
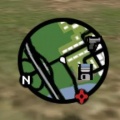
0xRRGGBB00
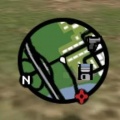
0xRRGGBB11
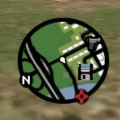
0xRRGGBB22
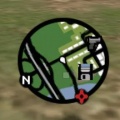
0xRRGGBB33
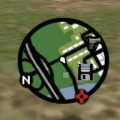
0xRRGGBB44
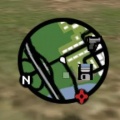
0xRRGGBB55
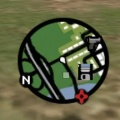
0xRRGGBB66
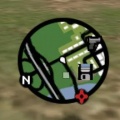
0xRRGGBB77
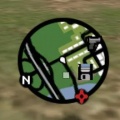
0xRRGGBB88
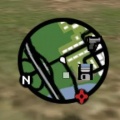
0xRRGGBB99
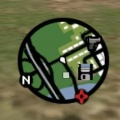
0xRRGGBBAA
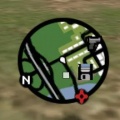
0xRRGGBBBB
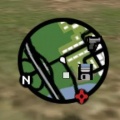
0xRRGGBBCC
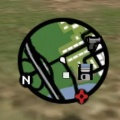
0xRRGGBBDD
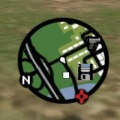
0xRRGGBBEE
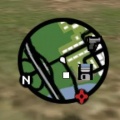
0xRRGGBBFF
Doing math
Since colors are just numbers it is possible to calculate with them, although it may not always make sense. For example, it is possible to adjust the player's radar marker visibility (see above) while keeping their current color the same, regardless of what is is.
SetPlayerMarkerVisibility(playerid, alpha = 0xFF) { new oldcolor, newcolor; alpha = clamp(alpha, 0x00, 0xFF); // if an out-of-range value is supplied we'll fix it here first oldcolor = GetPlayerColor(playerid); // get their color - Note: SetPlayerColor must have been used beforehand newcolor = (oldcolor & ~0xFF) | alpha; // first we strip of all alpha data (& ~0xFF) and then we replace it with our desired value (| alpha) return SetPlayerColor(playerid, newcolor); // returns 1 if it succeeded, 0 otherwise }
Convert string to value with pawn
In some cases, you might be interested in converting a hexadecimal string back into an integer. We've included an example function which allows you to do just that:
stock HexToInt(string[]) { if(!string[0]) return 0; new cur = 1, res = 0; for(new i = strlen(string); i > 0; i--) { res += cur * (string[i - 1] - ((string[i - 1] < 58) ? (48) : (55))); cur = cur * 16; } return res; }
You can call HexToInt to convert an RRGGBBAA string back into an integer.
Color embedding
It is possible to use colors within text in client messages, dialogs, 3D text labels, object material texts and vehicle numberplates.
It is very similar to gametext colors, but allows any color to be used.
It should be noted that this type of embedding won't work in textdraws. See this page for more information.
Example
{FFFFFF}Hello this is {00FF00}green {FFFFFF}and this is {FF0000}red
Another example

The code for the above chat line looks like this:
SendClientMessage(playerid, COLOR_WHITE, "Welcome to {00FF00}M{FFFFFF}a{FF0000}r{FFFFFF}c{00FF00}o{FFFFFF}'{FF0000}s {FFFFFF}B{00FF00}i{FFFFFF}s{FF0000}t{FFFFFF}r{00FF00}o{FFFFFF}!");
You can define colors to use like so:
#define COLOR_RED_EMBED "{FF0000}" SendClientMessage(playerid, -1, "This is white and "COLOR_RED_EMBED"this is red.");
..or
#define COLOR_RED_EMBED "FF0000" SendClientMessage(playerid, -1, "This is white and {"COLOR_RED_EMBED"}this is red.");
The second example would be better as is it clearer that embedding is used.
Using GetPlayerColor
To use a player's color as an embedded color, you must first remove the alpha value. To do this, perform a logical right shift.
new msg[128]; format(msg, sizeof(msg), "{ffffff}This is white and {%06x}this is the player's color!", GetPlayerColor(playerid) >>> 8); SendClientMessage(playerid, 0xffffffff, msg);
The %x is the placeholder for hexadecimal values, the 6 ensures that the output string will always be six characters long and the 0 will pad it with zeros if it's not.
Note that GetPlayerColor only works properly if SetPlayerColor has been used beforehand.
The colors used in color embedding are not like normal hex colors in Pawn. There is no '0x' prefix and no alpha value (last 2 digits).
Colour pickers
- SA-MP Colorpicker v1.1.0
- December.com
- RGB Picker
- Adobe Kuler
- Color Scheme Designer
- Google your RGB hexadecimal colour code, prepended with # (#RRGGBB)
GameText
Refer to this page for information about colour embedding with game texts.
Vehicle & ped colours
Vehicle and ped colours are different. There's a system in place which allows vehicle and ped developers to assign specific vehicle and ped colour sets to an individual ped or vehicle.
You don't have the freedom to use RGBA values - instead you will be working with a list of predefined colour IDs. We included a comprehensive list of these here:
Working with IDs
Working with these IDs is pretty straightforward - you can use any of the IDs defined on the above mentioned resource pages and you're good to go. The only thing to note is that there are a total of four different slots you can set - specific parts of an in-game model may be marked as "colourable". So, in other words, a vehicle can have a bonnet which is coloured by the secondary vehicle colour, while the rest of the vehicle is mapped to the primary vehicle colour. Of course, the bumpers aren't mapped to anything at all, and won't suddenly change colour just because you called ChangeVehicleColor. The same concept applies to the ped colour system, which is only available on GTA: Underground (specifically, on skins which actually have certain parts mapped to one of the four slots - as of Snapshot 4, only GTA: VCS skins use the ped colour feature!)
Example
This example changes the primary and vehicle colours of a vehicle.
ChangeVehicleColor(vehicleid, 275, 275);
Working with RGB values
Sometimes having a list of predefined vehicle or ped colours to choose from isn't enough. In some cases one might wish to use direct RGB vehicle colours rather than static IDs.
Switching RGB colours on and off
By default, RGB colours are disabled. This means that the game will always use the vehicle colour id which is applied to a vehicle, even after you call ChangeVehicleColorRGB. In order to turn RGB colours on or off on a specific vehicle, you have to use ToggleVehicleColorRGB. You'll have the option to turn RGB colours off on the primary, secondary, tertiary, and quaternary vehicle colour slot.
ToggleVehicleColorRGB(vehicleid, VEHICLE_COLOR_TERTIARY, true);
Changing the RGB colour of a vehicle
After you turned on RGB colours on your desired vehicle, you may call ChangeVehicleColorRGB like this:
ChangeVehicleColorRGB(vehicleid, VEHICLE_COLOR_TERTIARY, 255, 0, 0);
Extra weathers
Each in-game hour contains numerous RGB(a) colour values which have to be set when using extra weather types. This page contains more information about this.